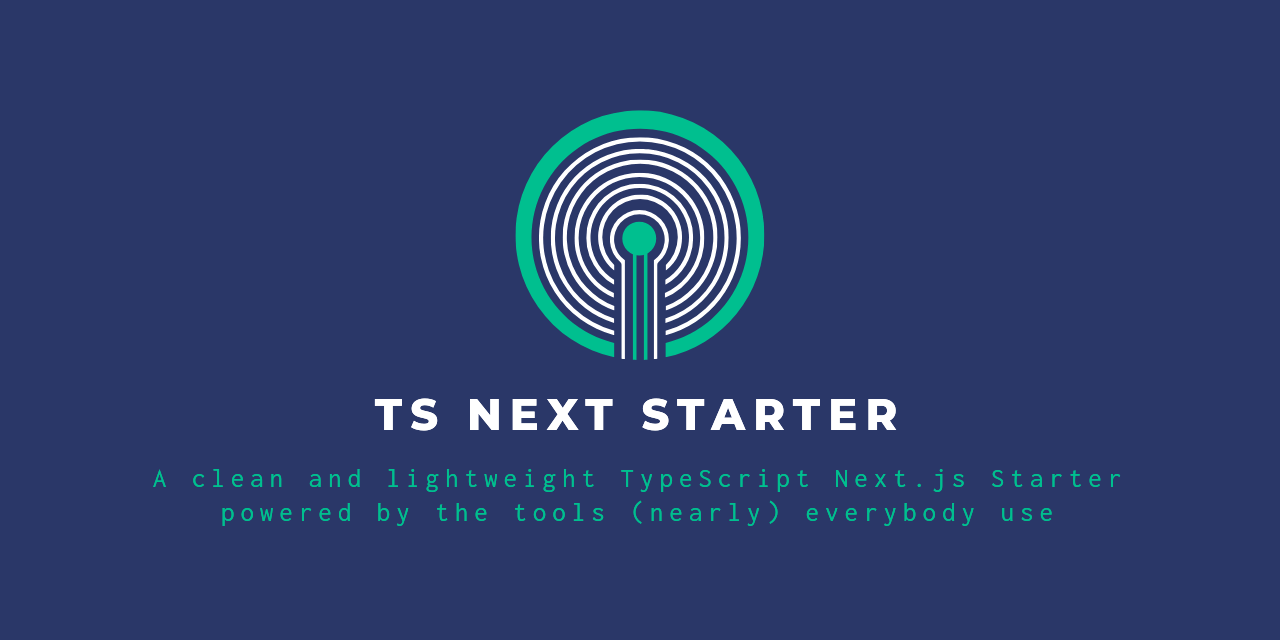
This is a template for a Next.js project based on create-next-app
Report Bug
·
Request Feature
⚡ Quickstart
You can click on the button Use this template or start a new project with create-next-app
npx create-next-app -e https://github.com/jmlweb/tsnextstarter
#or
yarn create next-app -e https://github.com/jmlweb/tsnextstarter
#or
pnpm create next-app -e https://github.com/jmlweb/tsnextstarter
🧠 Philosophy
- To be opinionated on the tools, but not on the code
- To help implementing a scalable, module-based directory organization
What's included
- The basic Next.js template with TypeScript support
- Jest and Testing Library (provided by @swc/jest for better performance)
- Mocking for http req and res (useful for API handlers)
- Two layouts are ready to be used: A "common" one (intended to be used as default) and an "empty" one (just in case you need a completely different look & feel for some pages)
- Storybook configured and integrated with Next.js
- Cypress is already configured for Next.js and integrated with GitHub actions
- prettier
- eslint
- prettier integration
- automatic import sorting
- Testing library integration
- conventional commits integration:
- linting
- commitizen integration
- changelog generation
- runs linting for code and commit message on each commit
- GitHub actions:
- linting
- testing & building
- package.json & tag version bumping (based on semantic commits)
What's not included?
- Any styling solution
- Any library for state management, nor for graphql/rest handling
- Visual changes to the standard create-next-app template
🎛️ Scripts
dev
: Start server on dev modebuild
: Build code for productionstart
: Start production serverlint
: Lint codetest
: Run Jesttest:watch
: Run Jest on watch mode for the file provided as argumenttest:watchAll
: Run Jest on watch mode for all the filestest:coverage
: Run Jest on coverage modeprepare
: Install husky hookscommit
: Run commitizenrelease
: Run changelog generation and version bumpingstorybook
: Run Storybook in development modebuild-storybook
: Run Storybook in build mode
🔪 Directories organization
src
For a better organization, the code is organized inside the src
folder, while the configuration files and the public
directory are located at the root level.
This is supported by Next.js by default.
src/pages
This folder is used only for routing purposes. Every file inside the pages
folder should not have any logic attached but to re-export the modules (default for the Component, named for server-side data fetching functions) from the scenes
folder.
src/scenes
A scene
represents a logical set of screens (or just one) from the application and is formed by:
- One or more "page" components.
- If they are needed, one or more data-fetching functions (
getStaticProps
,getStaticPaths
,getServerSideProps
) - Other files or folders which are used by the scene(s) component(s).
components
utils
*.stories.tsx
- …
Each scene
can serve to different pages
, by using different combinations of page components
and data-fetching functions
:
- A
scene
could export two different components (or "sub-scenes"), along with the samegetStaticProps
function. - The two components represent the same information and use internally most of the same sub-components while maintaining a completely different layout (including subtle variations on the sub-components).
- The route "/items/layout-a" could point to one of the components, while "/items/layout-b" could point to the other one.
If needed, a scene
can contain also other folders like components
, and data
… with modules shared across the scene.
If you want, you can place your unit tests and stories at the same level as your code.
src/apiHandlers
Each subfolder inside the apiHandlers
folder contains one or more handlers (which will be later imported by the api routes) and optionally the tests, utilities, or any other type of auxiliary file.
src/layouts
The layout is formed by a component, along with a function called getLayout
which will be imported by the scene and placed in the special getLayout
property of the scene component.
Refer to the Next.js documentation for more info about the layouts
src/components, src/data, src/helpers, src/utils…
Just use a folder at the src
level for any kind of file which is shared by two or more scenes
.
test
You can use this directory for your integration tests with Jest
cypress
e2e (and/or integration) tests and fixtures for Cypress
.storybook
Configuration files for Storybook
.github/workflows
CI/CD and bumping workflows for GitHub Actions
🤷 F.A.Q.
Shall I use the version bumping manually or with the action provided
If you are using already GitHub actions
- If are comfortable with the workflow, just remember not to run the
release
command manually - If you prefer to bump manually, delete the
bumping.yml
file inside.github/workflows
folder. Whenever you want to do the bumping, run therelease
script and push your files and tags.
If you are not using GitHub actions
You can safely delete the .github
folder or just forget about its existence as it won't harm your project.
How to integrate X feature/technology into my project?
Please, refer to the official Next.js documentation